Often we may need to pass variables from server side to client for using it in a
JavaScript
file or in a global script. It's possible to write JavaScript code from server side script, for example, we may use something like this:
echo "";
This will generate something like this:
So, we can use it in our
JavaScript
but if we want to pass an object we may pass it using similar approach by printing an object literal as an string, for example:
echo "";
It is like transforming a PHP
object into JavaScript
object from server to client side.
But, we can also use
Laravel View Composer
to accomplish same thing in a better way and we may pass an
Eloquent
model object as well, for example, if we want to pass the currently logged in user object to JavaScript as a
JavaScript
object, we may do this easily using a View
Composer
and can use it directly from any
JavaScript
segment in global scope or a
JavaScript
file.
Let's see an example, we'll create a
View Composer
for our
master layout
so every time we show our Website (using master template), the template will automatically get a user object in the
$user
variable and will dump it as a
json
string which will be available on the client side as a
JavaScript
object. Here is the code, we may keep it in our
filters.php
file:
View::composer('layouts.master', function($view) {
$user = null;
if(Auth::check()) {
$user = Auth::user();
}
$view->with('authUser', $user);
});
Well, we hust declared our View Composer and bound it to our master layout/template and now we'll use it on our template to generate a JavaScript object to use it later on client side:
That's it! Now, we can use the user object from our JavaScript code directly once the page is ready. So, now we can use our
authUser
object in any
JavaScript
code as an object.
Actually, when we echo the Eloquent model object in our blade template using
{{ $authUser or 'undefined' }}
the eloquent object become a
json string
because
Laravel
converts any
Eloquent
object or a collection of objects to a
json
string using
toJson()
method when we directly return it from any route, controller or print/echo it in our view. So, the output will be something like following if we use
console.log(authUser)
in the browser's console:
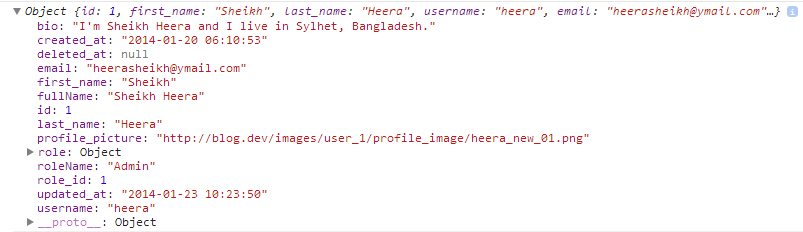
From our
View Composer
we are just passing the user object (currently logged in user) and in our template file we are printing it using
Blade Template
syntax, which is
{{ }}
, that's it. If we want to return a custom object from the View
Composer
we may pass a custom object but if it's not
Eloquent
model object then we may manually convert it to
json
string using
json_encode
function.
Update:
Often I see question asked on
StackOverflow
about how to use/generate a
URI
from
PHP
to use it in the client in a JavaScript file. This could be accomplished using this approach, for example to generate a
URI
for an
AJAX
request we can do something like this (create a view composer):
View::composer('layouts.master', function($view) {
$ajaxUrl = json_encode(array('url' => URL::action('getLocation')));
$view->with('ajax', $ajaxUrl);
});
This goes to master layout in the
head
section:
Now it's possible to use the
URI
in the client side using something like this:
$.ajax({
'url':ajax.url,
// ...
});